Reading integer streams and return N th order statistics
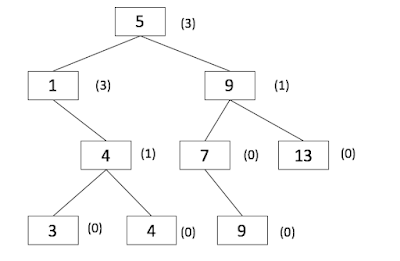
Problem Imagine you are reading in a stream of integers. Periodically, you wish to be able to look up the rank of a number x (the number of values less than or equal to x). Implement the data structures and algorithms to support these operations. The following methods need to be implemented. // method to take new number x void track(int x); // method to return the number of values that are less than or equal to x (not including x itself) int get_rank(int x); Example: Stream (in order of appearance): 5, 1, 4, 4, 5, 9, 7, 13, 3 get_rank(1) = 0 get_rank(3) = 1 get_rank(4) = 3