Decoding numeric string to alphabets.
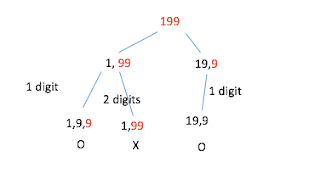
Problem Given a string of integers returns the number of ways to decode integers back to Alphabets using the following mapping: 'A' -> 1 'B' -> 2 ... 'Z' -> 26 examples: input = 199 output = 2 explanation: 1-> 9 -> 9 (AII) 11-> 9 (KI) input = 11 output = 1 explanation: 1->1 (AA) 11 (K)